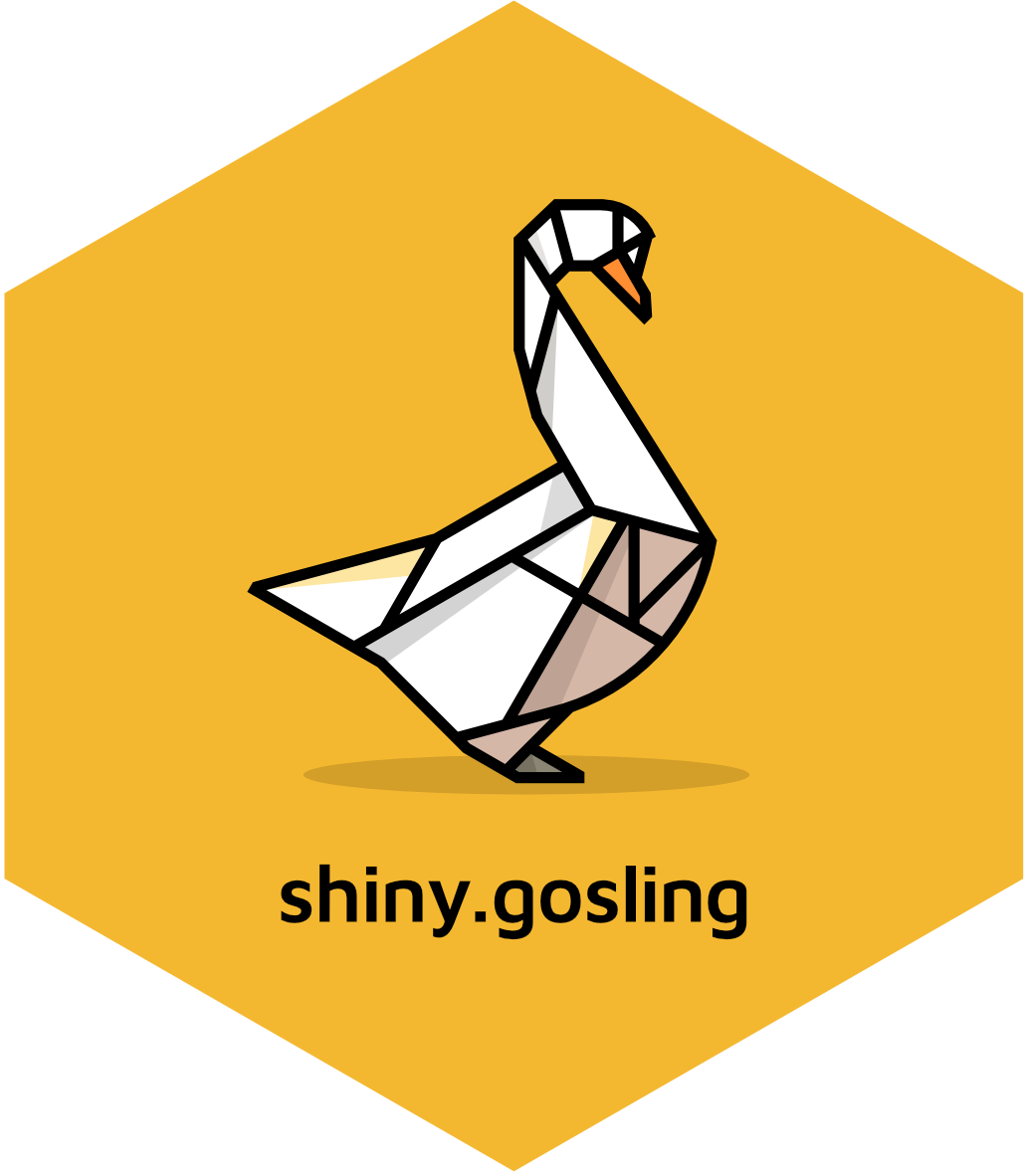
Data object builder for a csv file
track_data_csv.Rd
Build the data object for gosling plots
Usage
track_data_csv(
file,
genomicFields = NULL,
chromosomeField = NULL,
separator = ",",
sampleLength = 1000,
headerNames = NULL,
...
)
Arguments
- file
A character. Specify the URL address or local file name in the www directory of the data file.
- genomicFields
A character vector. Specify the name of genomic data fields.
- chromosomeField
A character. Specify the name of chromosome data fields.
- separator
A character. Specify file separator, Default: ','
- sampleLength
A number. Specify the number of rows loaded from the URL. Default: 1000
- headerNames
A character vector. Specify the names of data fields if a CSV file does not have header row.
- ...
Any other parameters passed to json data object.
Examples
if (interactive()) {
library(shiny.gosling)
library(shiny)
library(GenomicRanges)
url <- "https://rb.gy/7y3fx"
temp_file <- file.path(tempdir(), "GSM1295076_CBX6_BF_ChipSeq_mergedReps_peaks.bed.gz")
download.file(url, destfile = temp_file)
df <- read.delim(
temp_file,
header = FALSE,
comment.char = "#"
)
gr <- GRanges(
seqnames = df$V1,
ranges = IRanges(df$V2, df$V3)
)
if (!dir.exists("data")) {
dir.create("data")
}
utils::write.csv(gr, "data/ChipSeqPeaks.csv", row.names = FALSE)
ui <- fluidPage(
use_gosling(clear_files = FALSE),
goslingOutput("gosling_plot")
)
track_1 <- add_single_track(
width = 800,
height = 180,
data = track_data_csv(
"data/ChipSeqPeaks.csv", chromosomeField = "seqnames",
genomicFields = c("start", "end")
),
mark = "bar",
x = visual_channel_x(
field = "start", type = "genomic", axis = "bottom"
),
xe = visual_channel_x(field = "end", type = "genomic"),
y = visual_channel_y(
field = "width", type = "quantitative", axis = "right"
),
size = list(value = 5)
)
composed_view <- compose_view(
layout = "linear",
tracks = track_1
)
arranged_view <- arrange_views(
title = "Basic Marks: bar",
subtitle = "Tutorial Examples",
views = composed_view
)
server <- function(input, output, session) {
output$gosling_plot <- renderGosling({
gosling(
component_id = "component_1",
arranged_view
)
})
}
shiny::shinyApp(ui, server)
}